Front-end and Back-end Frameworks for Custom Software Development
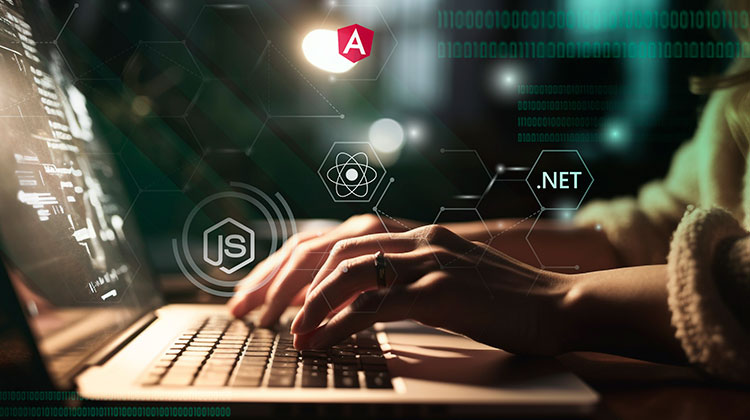
Building innovative, custom software applications involves two distinct yet interconnected layers: the front-end and the back-end.
The front-end is what users see and interact with—the user interface (UI). The back-end powers the application behind the scenes, handling data storage, server-side logic, and business processes. Together, they make up the tech stack of an application.
When a software engineer or custom software company talks about full-stack development, they’re talking about building both the front-end and back-end of a software product.
To streamline development in both these areas, developers often use frameworks. These pre-written code libraries and structures provide a foundation upon which applications can be built faster, cleaner, and with better maintainability.
Front-end Frameworks
Front-end frameworks make it easier to craft visually appealing, user-friendly interfaces. By providing pre-built components and functionalities, front end frameworks reduce the need to write code from scratch, accelerating the development process.
These frameworks enforce coding standards and best practices, leading to cleaner, more maintainable code across the development team. Standardized code structures and reusable components make it easier to manage and update the application, which facilitates collaborative development.
Many front-end frameworks are built with responsiveness in mind, ensuring the application adapts seamlessly to different screen sizes and devices.
React
React is a JavaScript library designed explicitly for building web application user interfaces (UI). Created by Facebook in 2013, it has since become one of the most popular and widely used front end frameworks in the world.
React applications are built from reusable components—independent, self-contained pieces of code that create a common function. This modular approach promotes code organization, maintainability, and reusability.
The React framework utilizes JSX, a syntax extension for JavaScript that allows developers to write HTML-like structures directly within JavaScript code. This makes it easier to visualize and think through the UI being built.
React employs a virtual Document Object Model (DOM)—an in-memory representation of the actual DOM of the web page. When changes occur in the application state, React efficiently updates the virtual DOM and determines the minimal set of changes required in the real DOM. This significantly improves rendering performance and the overall user experience.
The React framework follows a unidirectional data flow architecture, where data is passed down from parent components to child components in a single direction. This promotes better predictability and maintainability in an application’s state management.
Here are some of the benefits of using React:
- Faster development: By leveraging pre-built components and the efficient virtual DOM, React allows for faster development compared to traditional methods.
- Improved code quality: The component-based approach and JSX syntax encourage cleaner and more maintainable code.
- Enhanced developer experience: React offers a rich ecosystem of tools and libraries, along with a large and supportive community, making development more efficient and enjoyable.
- SEO advantages: React applications can be efficiently optimized for search engines, making them more discoverable.
Popular use cases for React include:
- Building single-page applications (SPAs)
- Creating interactive web interfaces
- Developing reusable UI components
- Building mobile applications with React Native
Overall, React is a powerful and versatile library for building modern, dynamic, and user-friendly web applications. Its component-based architecture, virtual DOM, and unidirectional data flow make it a popular choice for developers of all skill levels.
Angular
Angular is a comprehensive JavaScript framework used for building dynamic web applications, particularly single-page applications (SPAs). It was initially developed and released by Google in 2010 and has become a prominent choice for large-scale and complex web development projects.
The Angular framework adheres to the Model-View-Controller (MVC) pattern, which separates into distinct layers the application logic (Model), user interface (View), and Controller (handles user interactions and updates the View). The MVC pattern promotes code organization, maintainability, and testability.
Unlike React, which primarily uses JavaScript, Angular embraces TypeScript, a superset of JavaScript that adds optional static typing and other features. TypeScript helps enhance code readability and maintainability and helps catch errors early in the development process.
Angular also employs dependency injection—a design pattern that provides a controlled way of supplying dependencies to components and services—to improve code testability and modularity.
Finally, Angular comes packed with various built-in features like routing, forms management, HTTP client, and more, reducing the need for external libraries.
The benefits of using Angular include:
- Structured development: The MVC architecture and built-in features provide a clear structure and guide for development, especially in larger projects.
- Scalability: Angular is well-suited for building large and complex applications due to its robust features and structured approach.
- Improved code quality: TypeScript support and features like dependency injection contribute to cleaner, more maintainable, and testable code.
- Large community and resources: With a vast community and extensive ecosystem of libraries and tools, developers have access to ample support and learning resources.
Popular use cases for Angular include:
- Building single-page applications (SPAs)
- Developing enterprise-level web applications
- Creating complex and interactive web interfaces
- Building mobile applications with frameworks like Ionic or NativeScript
Angular is a powerful and versatile framework for building robust and scalable web applications. Its structured approach, comprehensive features, and large community make it a solid choice for developers seeking a well-defined framework for complex front-end development projects.
Vue
Vue is a progressive JavaScript framework used for building user interfaces (UI) and single-page applications (SPAs). Created by Evan You in 2014, Vue has gained significant popularity due to its ease of learning, flexibility, and performance.
Unlike front end frameworks that enforce a specific structure, Vue allows developers to gradually integrate its features into existing projects or start with a minimal setup. This flexibility makes it accessible to developers with varying experience levels.
Vue utilizes HTML-like templates to define the UI, making it easy for developers familiar with HTML to pick up quickly. These templates are enhanced with directives—special attributes that provide dynamic behavior and data binding.
The Vue framework employs a reactive system, meaning that when the underlying data changes, the UI automatically updates efficiently. This functionality simplifies development and ensures the UI always stays in sync with the data.
Vue’s core library is exceptionally lightweight, making it ideal for projects where performance is a critical concern. However, its rich ecosystem of libraries and tools allows developers to add functionalities as needed.
Some of Vue’s benefits include:
- Ease of learning: With its intuitive syntax and gradual adoption approach, Vue is considered easier to learn compared to other comprehensive frameworks like Angular. This low barrier to entry makes it attractive to beginners and experienced developers alike.
- Flexibility: Vue’s progressive nature allows developers to choose the level of complexity they need, making it suitable for small-scale projects to complex SPAs.
- Performance: The lightweight core and efficient reactivity system contribute to Vue’s strong performance, making it a good choice for applications requiring fast rendering and responsiveness.
- Versatility: Vue can be used in various scenarios, from building single-page applications and web UIs to creating interactive components and integrating with existing projects.
Popular use cases for Vue:
- Building single-page applications (SPAs)
- Developing web user interfaces
- Creating reusable UI components
- Integrating with existing projects (progressive adoption)
Vue offers a compelling option for front-end development, especially for those seeking a balance between ease of use, flexibility, and performance. Its approachable nature and progressive design make it a valuable tool for developers of all levels.
Bootstrap
Bootstrap is not a full-fledged framework like React, Angular, or Vue. Instead, it’s primarily a UI component library designed to simplify front-end development and make websites responsive across different devices.
Bootstrap offers a wide range of pre-designed and customizable components, including buttons, forms, navigation bars, modals, and more. This saves developers time and effort compared to building components from scratch.
One of Bootstrap’s core strengths is its focus on responsive design. It provides a grid system and utility classes that help developers easily adapt layouts and components to different screen sizes, ensuring optimal viewing experiences on desktops, tablets, and mobile devices.
Bootstrap utilizes basic HTML, CSS, and JavaScript, making it relatively easy to learn and integrate into existing projects. It also offers clear documentation and a large community for support.
The benefits of using Bootstrap include:
- Faster development: By leveraging pre-built components and responsive utilities, Bootstrap helps developers work more efficiently and reduce development time.
- Improved code consistency: Using Bootstrap’s established styles and components promotes consistency across the website’s design.
- Responsive design made easy: Bootstrap’s grid system and responsive utilities simplify the process of creating responsive layouts, ensuring a seamless user experience across different devices.
- Large community and resources: With a vast community and extensive documentation and tutorials, developers have ample resources for learning, troubleshooting, and finding solutions.
Popular use cases for Bootstrap:
- Building responsive websites quickly and efficiently
- Creating user interfaces with consistent styling
- Enhancing existing web projects with pre-built components
- Prototyping website designs with a basic layout structure
While Bootstrap simplifies development and offers responsive design features, it doesn’t provide the same level of structure and functionality as comprehensive frameworks like React, Angular, or Vue. These frameworks are better suited for building complex single-page applications with intricate features and functionalities.
Nevertheless, Bootstrap remains a valuable asset for front-end developers seeking to streamline their workflow, improve code consistency, and ensure responsive website design. Its ease of use, rich component library, and focus on responsiveness make it a popular choice for various web development projects.
Back-end Frameworks
Back-end frameworks allow a custom software company to manage server-side operations efficiently. These frameworks provide the mechanisms needed to connect to and interact with databases, making data storage and retrieval seamless.
Back-end frameworks have built-in security features that help protect applications from vulnerabilities and ensure secure user authentication and authorization.
These frameworks also simplify Application Programming Interface (API) development and management. APIs are essential for data exchange between different parts of the application or with external services.
Node.js
Node.js is not strictly a framework itself, but rather an open-source JavaScript runtime environment. It allows developers to execute JavaScript code outside of a web browser, enabling the creation of server-side applications and various other functionalities.
Node.js leverages the V8 JavaScript engine (the same engine that powers Google Chrome) to execute JavaScript code efficiently. This allows developers to utilize familiar JavaScript syntax and libraries on the server side.
Unlike traditional web servers that create separate threads for each request, Node.js employs an event-driven, non-blocking I/O model. This means it handles multiple requests concurrently without blocking the main thread, making it efficient for handling numerous concurrent connections effectively.
Node.js comes bundled with Node Package Manager (npm), a vast repository of open-source JavaScript packages that provide pre-built modules and functionalities for various development needs. This extensive ecosystem empowers developers to leverage existing solutions and accelerate development.
Benefits of using Node.js:
- Faster development: By enabling JavaScript code execution on both the front-end and back-end, Node.js streamlines development by allowing developers to use a single language throughout the stack, potentially reducing context switching and improving development efficiency.
- Scalability and performance: The event-driven, non-blocking I/O model makes Node.js highly scalable, allowing it to handle a large number of concurrent connections efficiently. This is particularly beneficial for real-time applications and data-intensive tasks.
- Rich ecosystem of libraries: With a vast and ever-growing ecosystem of packages available through npm, developers can find solutions for various functionalities, reducing the need to develop everything from scratch.
- Familiar language for front-end and back-end: For developers already familiar with JavaScript, Node.js offers a smooth transition to server-side development, leveraging their existing knowledge and skillset.
Popular use cases for Node.js:
- Building real-time applications (e.g., chat applications, online games)
- Developing APIs (Application Programming Interfaces)
- Creating microservices architectures
- Implementing server-side scripting
- Building command-line tools
Node.js has established itself as a versatile and powerful runtime environment for modern JavaScript development. Its ability to leverage JavaScript across the front-end and back-end, combined with its scalability, rich ecosystem, and efficient I/O model, make it a compelling choice for various application types and development needs.
Express.js
Express.js, often simply referred to as Express, is a minimalist, fast, and web-focused framework built on top of Node.js. It provides a flexible foundation for building various web applications and APIs. Unlike some frameworks that enforce a specific structure, Express allows for a more modular and customizable approach to development.
Express boasts a lightweight core, making it ideal for projects where performance is a critical concern. This allows developers to choose the functionalities they need and avoid unnecessary overhead.
Express offers a high degree of flexibility and customization. Developers can choose from various middleware functions and libraries to create the desired functionality for their applications.
One of the core functionalities of Express is routing, which allows developers to define how the application responds to different URL requests (endpoints).
Express also features middleware functions—reusable blocks of code that handle requests and responses before they reach the final route handler. They can be used for various tasks like authentication, logging, or data parsing.
Benefits of using Express.js:
- Faster development: The minimalist approach and modularity of Express allow developers to get started quickly and build applications efficiently.
- Performance: The lightweight core contributes to the performance of applications built with Express, making it suitable for real-time applications and high-traffic websites.
- Flexibility and customization: Developers have considerable control over the application’s structure and functionality, enabling them to tailor it to specific needs.
- Large community and ecosystem: Express boasts a vast and active community that provides extensive documentation, tutorials, and middleware libraries, simplifying development and troubleshooting.
Popular use cases for Express:
- Web applications (single-page and multi-page)
- RESTful APIs
- Real-time applications (e.g., chat applications)
- Proxy servers
Express.js is often used in conjunction with other frameworks or libraries like React or Vue.js for front-end development or database management libraries like Mongoose.
Express.js stands out as a versatile and powerful framework for building web applications and APIs in Node.js. Its lightweight core, flexibility, and extensive ecosystem make it a valuable tool for developers seeking to create efficient and customizable applications.
.NET
Microsoft’s .NET encompasses a broad range of development tools, languages, libraries, and frameworks created and maintained by Microsoft. It’s designed to simplify and streamline the process of building various types of software applications.
The two core components of .NET are the Common Language Runtime (CLR) and the .NET Framework.
The foundation of the .NET platform, the CLR is a virtual machine that executes code written in different .NET-compatible languages. It provides functionalities like memory management, security, and garbage collection, allowing developers to focus on application logic.
The .NET Framework is the original implementation of .NET, primarily targeting Windows development. It offers a vast library of classes and functionalities for building various types of applications, including desktop apps, web services, and more. However, its development has largely transitioned to .NET.
.NET is the modern and cross-platform successor to the .NET Framework. It embraces open-source principles and supports development for various operating systems like Windows, Linux, and macOS.
.NET Core is a subset of .NET, focusing on building lightweight and modular applications. It serves as the foundation for many other components within the .NET ecosystem.
Key benefits of using .NET:
- Cross-platform development: Unlike the .NET Framework, .NET allows developers to build applications for various platforms, providing greater flexibility and reach.
- Rich ecosystem: .NET boasts a vast ecosystem of libraries, frameworks, and tools, empowering developers to find solutions for diverse development needs.
- Strong community and support: Microsoft and a large community actively support and contribute to the .NET ecosystem, providing extensive documentation, resources, and assistance.
- Mature and feature-rich: With a long history and continuous development, .NET offers a mature and feature-rich platform for building various application types.
Popular use cases for .NET:
- Building web applications (ASP.NET)
- Developing mobile applications (Xamarin)
- Creating desktop applications (WPF, Windows Forms)
- Implementing cloud-native applications
- Building microservices architectures
The .NET ecosystem provides a comprehensive and versatile platform for building software applications. Its cross-platform capabilities, rich ecosystem, and strong community support make it a compelling choice for developers seeking a robust and well-established development environment.
Django
Django is a high-level Python web framework widely used for developing complex and dynamic web applications. Created by Adrian Holovaty and others in 2005, Django is known for its “batteries-included” philosophy, meaning it comes equipped with a wide range of features out of the box, allowing for rapid development.
Django adheres to the Model-View-Template (MVT) architecture, which separates the application logic (models), user interface (views), and presentation layer (templates) into distinct layers. MVT promotes code organization, maintainability, and testability.
The Django framework provides a powerful ORM that simplifies interactions with relational databases. Developers can work with databases using Python objects, making data access and manipulation more intuitive.
Django offers a built-in administrator interface that allows for easy management of models, users, and content within the application. This is particularly useful for managing data and content without needing to build a separate administrative interface from scratch.
When it comes to security, Django includes various built-in features to prevent common web vulnerabilities like cross-site scripting (XSS) and SQL injection.
Benefits of using Django:
- Rapid development: The “batteries-included” approach and features like the ORM and admin interface significantly accelerate the development process compared to building applications from scratch.
- Reusability and maintainability: Django encourages code reuse and promotes clean and maintainable code through features like models and views.
- Security: Django’s built-in security features help developers avoid common pitfalls and create secure web applications.
- Large community and resources: With a vast and active community, Django offers extensive documentation, tutorials, and support, making it easier to learn and troubleshoot issues.
Popular use cases for Django:
- Building complex web applications
- Developing e-commerce platforms
- Creating content management systems (CMS)
- Implementing social networking applications
Overall, Django remains a popular choice for building robust and secure web applications in Python. Its comprehensive features, rapid development approach, and strong community support make it a valuable tool for developers of various skill levels.
Laravel
Laravel is a free and open-source PHP web framework designed to streamline the development process for building modern and dynamic web applications. Created by Taylor Otwell in 2011, Laravel has gained significant popularity due to its extensive features, expressive syntax, and vast ecosystem.
Like many other back-end frameworks, Laravel adheres to the MVC architecture, which separates concerns and promotes code organization, maintainability, and testability.
Laravel’s built-in ORM, Eloquent, simplifies database interactions by allowing developers to work with databases using PHP objects. This makes data access and manipulation more intuitive and efficient.
The Laravel framework includes the Blade templating engine, which offers a clean and expressive way to define the application’s views. Blade templates integrate seamlessly with PHP code, allowing for easy logic integration within the presentation layer.
Laravel includes a powerful Artisan command-line interface that provides various helpful functionalities like generating code, running migrations, and managing database seeding.
Laravel prioritizes security and incorporates various built-in features to protect against common web vulnerabilities like SQL injection and cross-site scripting (XSS).
Benefits of using Laravel:
- Rapid development: The comprehensive features and tools like Eloquent, Blade, and Artisan significantly accelerate development compared to building applications from scratch.
- Expressive and elegant syntax: Laravel’s syntax is designed to be clear, concise, and readable, making development more enjoyable and maintainable.
- Security focus: Built-in security features help developers create secure applications by mitigating common security risks.
- Large community and ecosystem: Laravel boasts a vast and active community that provides extensive documentation, tutorials, and packages, making it easier to learn and find solutions for development needs.
Popular use cases for Laravel:
- Building complex web applications
- Developing e-commerce platforms
- Creating content management systems (CMS)
- Implementing social networking applications
Laravel stands out as a powerful and expressive framework for building robust and secure web applications in PHP. Its comprehensive features, rapid development tools, and strong community support make it a popular choice for developers seeking a productive and enjoyable development experience.
Ruby on Rails
Ruby on Rails is a high-level web application framework built on top of the Ruby programming language. It’s renowned for its convention-over-configuration (CoC) approach, which streamlines development by providing default configurations and structures, allowing developers to focus on writing the core application logic.
Ruby on Rails adheres to the MVC architecture, which separates the application logic (models), user interface (views), and controller (handles user interactions) into distinct layers. This promotes code organization, maintainability, and testability.
Ruby onRails follows a CoC approach, meaning it assumes certain conventions for code structure and configuration. This approach reduces the amount of boilerplate code developers need to write, accelerating development.
Ruby on Rails provides a powerful ORM called Active Record. It simplifies database interactions by allowing developers to work with databases using Ruby objects, making data access and manipulation more intuitive.
The Ruby on Rails framework comes with various built-in features like scaffolding, routing, generators, and more, which further reduce development time and effort.
Benefits of using Ruby on Rails:
- Rapid development: The CoC approach, features like Active Record, and generators significantly accelerate the development process compared to building applications from scratch.
- Focus on business logic: By handling boilerplate tasks and conventions, Rails allows developers to focus on writing the core functionality and business logic of the application.
- Large and active community: Rails boasts a vast and active community that provides extensive documentation, tutorials, and support, making it easier to learn and find solutions for development challenges.
- Scalability: While initially known for rapid development, Rails applications can be scaled effectively to handle growing user bases and application complexity.
Popular use cases for Ruby on Rails include building web applications of various types, including:
- E-commerce platforms
- Content management systems (CMS)
- Social networking applications
- SaaS (Software as a Service) applications
- Prototyping and minimum viable products (MVPs)
Overall, Ruby on Rails remains a popular choice for building web applications due to its rapid development approach, convention-over-configuration style, and strong community support. It empowers developers to focus on the core functionality of the application while providing a robust and scalable foundation.
Building a Solid Foundation
Choosing the right frameworks can significantly impact the success of a custom software development project.
By considering project requirements, team capabilities, and future needs, a custom software company can set the foundation for building robust, efficient, and user-friendly applications.
If you need help with front-end, back-end, or full-stack development, contact the experts at Taazaa.